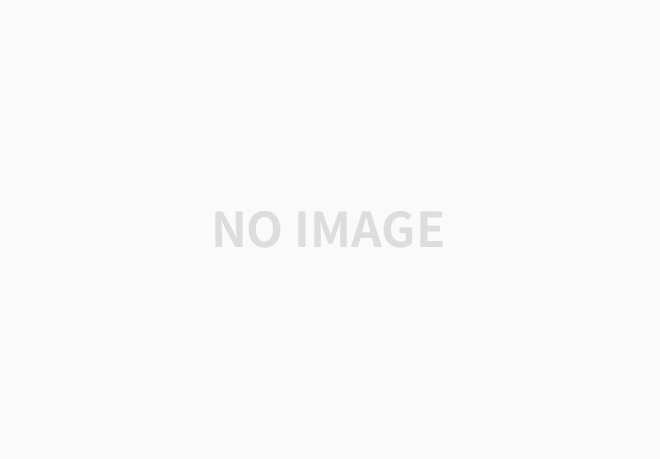
URL: https://docs.oracle.com/javase/7/docs/api/java/lang/Object.html
Object (Java Platform SE 7 )
Called by the garbage collector on an object when garbage collection determines that there are no more references to the object. A subclass overrides the finalize method to dispose of system resources or to perform other cleanup. The general contract of fi
docs.oracle.com
모든 클래스의 최상위 클래스
java.lang.Object 클래스
-모든 클래스는 Object 클래스에서 상속 받음
-모든 클래스는 Object 클래스의 메서드를 사용 할 수 있음
-모든 클래스는 Object 클래스의 일부 메서드(Final)로 정의된 클래스는 하위클래스에서 재정의를 한 것을 제외하고
재정의하여 사용할 수 있음
object 클래스 Source Code
package object;
class Book{
String title;
String author;
public Book(String title,String author) {
this.title = title;
this.author = author;
}
@Override
public String toString() {
return author+","+title;
}
}
public class ToStringTest {
public static void main(String[] args) {
Book book = new Book("토지","박경리");
//현재 해쉬코드 메모리의 주소가 나옴
System.out.println(book);
//String 안에 to String에 포함되어 있어서 가능하다.
//어떤 객체의 정보를 스트링형태로 표현해야할때 사용되는 메소드
String str = new String("토지");
System.out.println(str.toString());
}
}
toString() 메서드
- 객체의 정보를 String으로 바꾸어 사용할때 유용함
- 자바 클래스중에는 이미 구현되어 있는 클래스가 많다.
- ex) String, Integer, Calendar 등
- 많은 클래스 상에서 재정의하여 사용됨
->getClass().getName()+'@'+Integer.toHexString(hashCode())
equals() 메서드
- 두 객체(인스턴스)의 동일함을 논리적으로 재정의 할 수 있다.
- 물리적 동일함: 같은 주소를 가지는 객체
- 논리적 동일함: 같은 학번의 학생, 같은 주문 번호의 주문
-> 물리적으로 다른 메모리에 위치한 객체라도 논리적으로 동일함을 구현하기 위해 사용하는 메서드
Student studentLee = new Student(200,"박주상");
Student studentLee2 = studentLee;
Student studentSang = new Student(100,"이삭도");
물리적으로 다른 위치에 잇지만, 논리적으로는 같은 학생임을 구현
package object;
class Student{
int studentNum;
String studentName;
public Student(int studentNum,String studentName) {
this.studentNum=studentNum;
this.studentName=studentName;
}
//학번 사번 등의 같을 경우의 처리에 따른 작업을 할떄 필요하다.
@Override
public boolean equals(Object obj) {
// return super.equals(obj);
if(obj instanceof Student) {
Student std = (Student)obj;
// return (this.studentNum==std.studentNum);
//디테일하게 하려면 아래로 수정
if(this.studentNum==std.studentNum)
return true;
else return false;
}
return false;
}
}
public class EqualsTest {
public static void main(String[] args) {
String str1 = new String("abc");
String str2 = new String("abc");
//메모리 주소의 같은 경우의 여부를 확인->false
System.out.println(str1==str2);
//문자열이 같으면 같은 값을 나타내므로 -> true
System.out.println(str1.equals(str2));
Student Lee = new Student(100,"이순신");
Student Lee2 = Lee;
Student Shin = new Student(100,"이순신");
//true
System.out.println(Lee == Lee2);
//false->인스턴스 주소가 다르므로
System.out.println(Lee == Shin);
//논리적으로 같다라는 것을 재정의한 것 1. false 위의 오버라이드 구현 후에는 true
System.out.println(Lee.equals(Shin));
//hashCode->십진수의 값을 반환-> 두개의 값을 비교하면 값이 다르다.
System.out.println(Lee.hashCode());
System.out.println(Shin.hashCode());
//hashCode() 메서드의 반환 값 : 인스턴스가 저장된 가상 머신의주소를 10진수로 반환
// 두개의 서로 다른 메모리에 위치한 인스턴스가 동일하다는 것은
// 논리적으로 동일한 경우 : equals() 의 반환값이 true
// 동일할 hashCode 값을 가짐: hashCode()의 반환값이 동일
//실제 메모리의 주소는 동일 하고 -> 해쉬코드를 단지 오버라이딩
}
}
clone() 메소드
- 객체의 복사본을 만듦
- 기본 틀(protype)으로 부터 같은 속성 값을 가진 객체의 복사본을 생성할 수 있음
- 객체지향 프로그래밍의 정보은닉에 위배되는 가능성이 있으므로 복제할 객체는 cloneable 인터페이스를 명시해야함
- 생성자랑은 다른 개념 생성자는 이니셜라이져 하면서 만드는 것이고 클론의 경우에는 인스턴스의 지금의 상태를 복제 하는 것이다.
'JAVA' 카테고리의 다른 글
List 인터페이스 (0) | 2019.10.08 |
---|---|
제네릭 (0) | 2019.10.07 |
컬렉션 프레임워크(Collection Framework) (0) | 2019.10.06 |
String 클래스 (0) | 2019.10.05 |
인터페이스(Interface) (0) | 2019.10.02 |