오류란 ?
- 컴파일 오류 : 프로그램 코드 작성중 발생하는 문법적 오류
- 실행 오류 : 실행중인 프로그램이 의도하지 않은 동작을 하거나(bug) 프로그램이 중지 되는 오류 (runtime error)
자바는 예외 처리를 통하여 프로그램의 비정상 종료를 막고 log를 남길 수 있음
오류와 예외 클래스
- 시스템 오류(error) : 가상 머신에서 발생, 프로그래머가 처리 할 수 없음.
동적메모리를 다 사용한 경우, stack over flow등
- 예외( Exception) : 프로그램에서 제어 할 수 있는 오류
읽으려는 파일이 없는 경우, 네트웍이나 소켓 연결 오류 등
자바 프로그램에서는 예외에 대한 처리를 수행 함
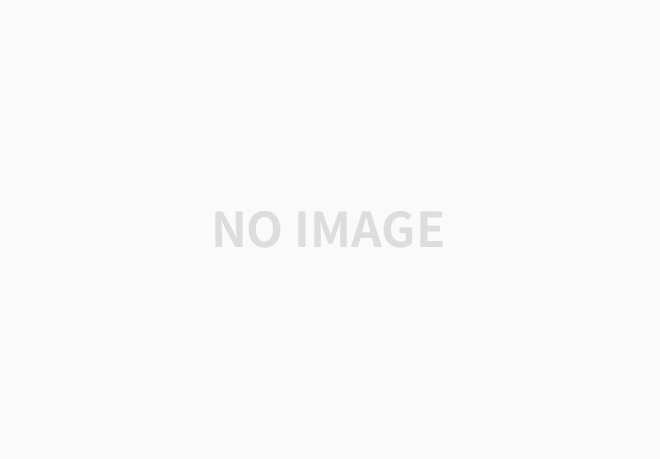
예외 클래스
- 모든 예외 클래스의 최상위 클래스는 Exception 클래스
try-catch 문으로 예외처리하기
try {
예외가 발생 할 수 있는 코드 부분
}catch (처리할 예외 타입 e){
try블록 안에서 예외가 발생했을 때 수행되는 부분
} finally{
예외 발생 여부와 상관 없이 향상 수행되는 부분
리소스를 정리하는 코드를 주로씀
}
Code
package exception;
public class ArrayExceptionTest {
public static void main(String[] args) {
int[] arr = new int[5];
try {
for(int i = 0; i<=5;i++) {
System.out.println(arr[i]);
}
}catch(ArrayIndexOutOfBoundsException e) {
System.out.println(e.toString());
System.out.println("예외처리");
}
System.out.println("프로그램종료");
}
}
package exception;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class ExceptionTest {
public static void main(String[] args) {
FileInputStream fis =null;
try {
fis = new FileInputStream("a.txt");
} catch (FileNotFoundException e) {
System.out.println(e);
return;
}finally {
try {
fis.close();
System.out.println("finally");
} catch (IOException e) {
System.out.println(e);
}
System.out.println("end");
}
}
}
try-with-resource 문
- 리소스를 자동으로 해제 하도록 제공해주는 구문
- 해당 리소스가 AutoCloseable을 구현한 경우 close()를 명시적으로 호출하지 않아도
try{} 볼록에서 오픈된 리소스는 정상적인 경우나 예외가 발생한 경우 모두 자동으로close()가 호출됨
- 자바7부터 제공
- FileInputStream의 경우 AutoCloseable을 구현 하고 있음.
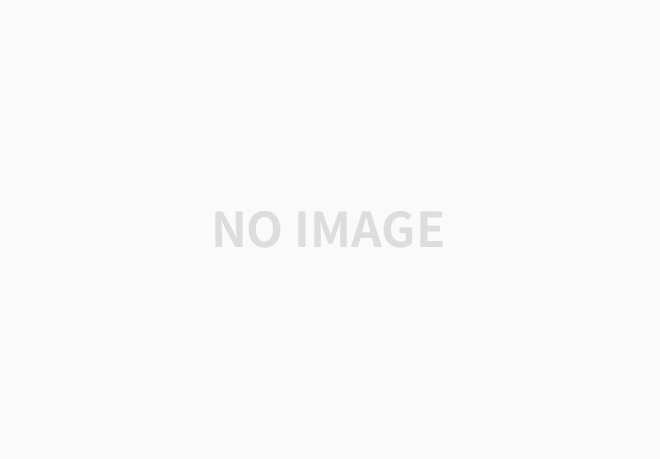
향상된 try-with-resource문
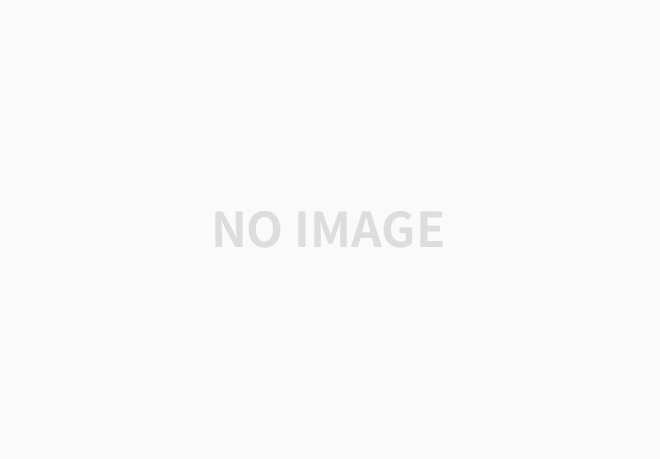
예외 처리 미루기
- throws 를 사용하여 예외처리 미루기
- try{} 블록으로 예외를 처리하지 않고, 메서드 선언부에 throws를 추가
- 예외가 발생한 메서드에서 예외 처리를 하지 않고 이 메서드를 호출한 곳에서 예외처리를 한다는 의미
- main()에서 throws를 사용하면 가상머신에서 처리 됨
다중 예외 처리하기
- 하나의 try{} 블록에서 여러 예외가 발생하는 경우 cathc{} 블록 한곳에서 처리하거나 여러 catch{}블록으로 나누어 처리 할 수 있음
-가장 최상위 클래스인 Exception 클래스는 가장 마지막 블록에 위치 해야함
ex) exception e 마지막에 놓고 사용
package exception;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class ThrowsException {
public Class loadClass(String fileName,String className) throws FileNotFoundException,ClassNotFoundException {
FileInputStream fis = new FileInputStream(fileName);
Class c = Class.forName(className);
return c;
}
public static void main(String[] args) {
ThrowsException test = new ThrowsException();
try {
test.loadClass("a.txt", "java.lang.string");
} catch (FileNotFoundException e) {
System.out.println(e);
}catch(ClassNotFoundException e){
System.out.println(e);
}catch(Exception e){
System.out.println(e);
}
}
}
사용자 정의 예외처리 방법
- JDK에서 제공되는 예외 클래스 외에 사용자가 필요에 의해 예외 클래스를 정의하여 사용
- 기존 JDK클래스 에서 상속받아 예외 클래스를 만듬
- throw 키워드로 예외를 발생시킴
package exception;
public class IDformatTest {
private String userID;
public String getUserID() {
return userID;
}
public void setUserID(String userID) throws IDFormatException {
if(userID==null) {
throw new IDFormatException("아이디는 null 일수 없습니다.");
}
else if (userID.length()<8||userID.length()>20) {
throw new IDFormatException("아이디는 8자 이상 20자 이하로 쓰세요");
}
this.userID = userID;
}
public static void main(String[] args) {
IDformatTest idTest = new IDformatTest();
String myId = null;
try {
idTest.setUserID(myId);
} catch (IDFormatException e) {
System.out.println(e);
}
}
}
================================================================
package exception;
public class IDFormatException extends Exception{
public IDFormatException(String message) {
super(message);
}
}
'JAVA' 카테고리의 다른 글
자바 입출력 스트림 (0) | 2019.10.18 |
---|---|
Multi-Thread 프로그래밍 (0) | 2019.10.17 |
스트림관련 코딩 (0) | 2019.10.16 |
스트림(Stream) (0) | 2019.10.15 |
람다식 (0) | 2019.10.14 |